추천할 만한 java design pattern 사이트
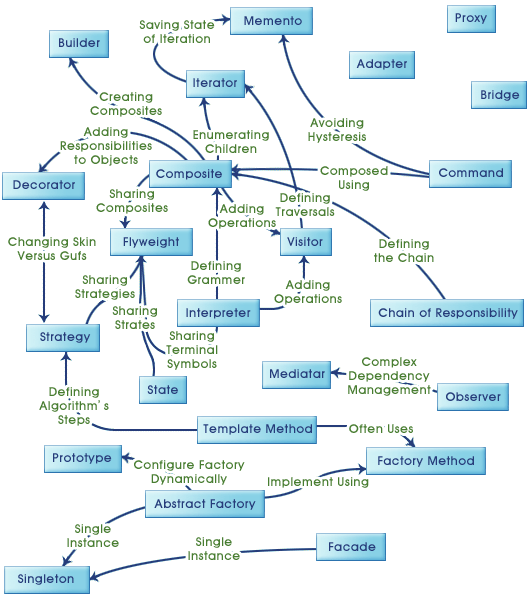
Creational Design Patterns:Click to zoom
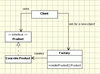 Factory(Simplified version of Factory Method) - Creates objects without exposing the instantiation logic to the client and Refers to the newly created object through a common interface. When to Use , Common Usage Click to zoom
Document Application Example
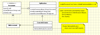 Factory Method - Defines an interface for creating objects, but let subclasses to decide which class to instantiate and Refers to the newly created object through a common interface. When to Use , Common Usage Click to zoom
Text Converter Example
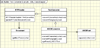 Builder - Defines an instance for creating an object but letting subclasses decide which class to instantiate and Allows a finer control over the construction process. Example: Text Converter in Java Click to zoom
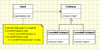 Prototype - Specify the kinds of objects to create using a prototypical instance, and create new objects by copying this prototype. Behavioral Design Patterns:Click to zoom
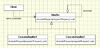 Chain of Responsibiliy - It avoids attaching the sender of a request to its receiver, giving this way other objects the possibility of handling the request too. - The objects become parts of a chain and the request is sent from one object to another across the chain until one of the objects will handle it. Sourcecode: Click to zoom
Restaurant Example
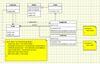 Command - Encapsulate a request in an object, Allows the parameterization of clients with different requests and Allows saving the requests in a queue. Sourcecode: Buying/Selling stocks in Java Click to zoom
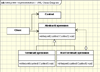 Interpreter - Given a language, define a representation for its grammar along with an interpreter that uses the representation to interpret sentences in the language / Map a domain to a language, the language to a grammar, and the grammar to a hierarchical object-oriented design Sourcecode: Romans Numerals Converter in Java Click to zoom
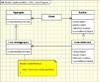 Iterator - Provide a way to access the elements of an aggregate object sequentially without exposing its underlying representation. Sourcecode: Java Iterator Click to zoom
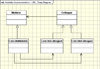 Mediator - Define an object that encapsulates how a set of objects interact. Mediator promotes loose coupling by keeping objects from referring to each other explicitly, and it lets you vary their interaction independently. Sourcecode: Click to zoom
News Publisher Example
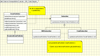 Observer - Define a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. Sourcecode: News Publisher in Java Click to zoom
Robot Example
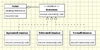 Strategy - Define a family of algorithms, encapsulate each one, and make them interchangeable. Strategy lets the algorithm vary independently from clients that use it. Sourcecode: Robot Application in Java Click to zoom
Travel Example
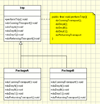 Template Method - Define the skeleton of an algorithm in an operation, deferring some steps to subclasses / Template Method lets subclasses redefine certain steps of an algorithm without letting them to change the algorithm's structure. Sourcecode: Travel Agency Application in Java Click to zoom
Customers Example
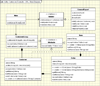 Visitor - Represents an operation to be performed on the elements of an object structure / Visitor lets you define a new operation without changing the classes of the elements on which it operates. Sourcecode: Customers Report Example Click to zoom
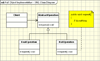 Null Object - Provide an object as a surrogate for the lack of an object of a given type. / The Null Object Pattern provides intelligent do nothing behavior, hiding the details from its collaborators. Sourcecode: Structural Design Patterns:Click to zoom
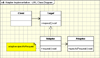 Adapter - Convert the interface of a class into another interface clients expect. / Adapter lets classes work together, that could not otherwise because of incompatible interfaces. Click to zoom
Click to zoom
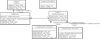 Bridge - Compose objects into tree structures to represent part-whole hierarchies. / Composite lets clients treat individual objects and compositions of objects uniformly. Sourcecode: Object Persistence Api in Java Click to zoom
Shapes Example
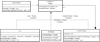 Composite - Compose objects into tree structures to represent part-whole hierarchies. / Composite lets clients treat individual objects and compositions of objects uniformly. Sourcecode: Shapes Example in Java Click to zoom
Wargame Example
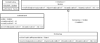 Flyweight - use sharing to support a large number of objects that have part of their internal state in common where the other part of state can vary. Sourcecode: Java Wargame Example Click to zoom
Calculator Example
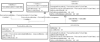 Memento - capture the internal state of an object without violating encapsulation and thus providing a mean for restoring the object into initial state when needed. Source Code: Calculator Example in Java Click to zoom
Image Viewer
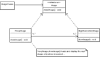 Proxy - provide a “Placeholder” for an object to control references to it. Sourcecode: Proxy Pattern in Java |